BoxFormBuilder
Introduction
BoxFormBuilder is a support class for the construction of Swing forms. Building Swing user interface often poses visual appearance problems to resolve resulting in a cumbersome code, whether programmed manually, or if you use an graphic editor of forms.
BoxFormBuilder allows the creation of professional looking forms, that supports size changes with a simple and clear code.
BoxFormBuilder uses exclusively BoxLayouts for the building of forms.
BoxFormBuilder is in the library jdal-swing since version 1.0, but has developed en two classes, since version 1.2.1. The class SimpleBoxFormBuilder which comes directly from BoxFormBuilder original and allows the creation of simple forms and the new BoxFormBuilder which allows the creation of complex components by the nesting of the simple components.
SimpleBoxFormBuilder
SimpleBoxFormBuilder constructs the Swing interfaces by the repitition of a basic template formded by the unión of a HorizontalBox and various VerticalBox to create a tabular struct.
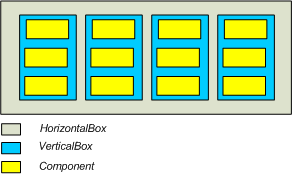
SimpleBoxFormBuilder adds components to this structure by the use of an implicit cursor. The Swing components are added to the form by the methods add(Component c) for individual components and addComponent(String label, Component c) to include at the same time a Jlabel and a Component. The component is added to the place pointed to by an internal cursor of SimpleBoxFormBuilder and it increases automatically in the calls to the methods add().
To change the column call the method row() that permits to specify the maximum height of the line, that will be added. The value by default is 25 points. It´s possible that you have to adjust the value, depending on the Look & Feel that is used.
BoxFormBuilder
In the above figure, the yellow place can be occupied directly by a Swing component or by a new basic template created by a new SimpleBoxFormBuilder. This model of composition gives place to a flexible system that practically covers the total distribution of usual components in the graphic user interfaces.
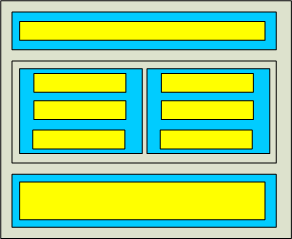
BoxFormBuilder adds the methods startBox() and endBox() to the class SimpleBoxFormBuilder to permit the addition of a new component in the place pointed at by the internal cursor. From the call to startBox() the subsequent calls to methods add() and row() are redirected to a new instance of SimpleBoxFormBuilder. The method endBox() adds the result to the position identified in the origianl compinent and restore the original builder.
The figure on the left shows the distribution of the components of a form formed by a JComboBox, three JTextFields preceeded by labels and a JTexArea. I recommend that before you start the creation of a form with LeBoxFormBuilder do a small drawing on a paper, clearly identifying the components that it is made up from. In this case there are three basic components distributed in just one column. The first two rows which JComboBox and the JTextFields have a fixed height. The last row, which contains the text area, has a variable size variable on the component size which contains the form. By changing the size of the form, the extra space will go to the text area.
The method setDebug(boolean debug) shows the borders of the BoxLayouts which are used in the construction of the forms which are useful during the construction of the same. Finally the method setFixedHeight(boolean fixed) permits the restriction of the height of the components created in the method startBox().
Below is the code to build the form.
/** * Sample for BoxFormBuilder * * @author Jose Luis Martin */ public class SampleForm extends JDialog { private JComboBox sex = new JComboBox(); private JTextField name = new JTextField(); private JTextField surname = new JTextField(); private JTextField secondSurname = new JTextField(); private JTextArea description = new JTextArea(); public SampleForm() { this.add(build()); } private JComponent build() { // create a box form builder and set a titled border in the created component. BoxFormBuilder fb = new BoxFormBuilder(BorderFactory.createTitledBorder(("Sample"))); fb.row(); // start a new base component row fb.startBox(); // add new simple box fb.setFixedHeight(true); // fix the height of this simple box fb.row(); // add row, now in the simple box. fb.add(sex); // add the sex combo; fb.endBox(); // end the simpleBox; fb.row(); // add new row in base component. fb.startBox(); // add new simpleBox fb.setFixedHeight(true); // fix the height of this simple box fb.row(); // add new row in the new simpleBox fb.add("Nombre: ", name); // add the name text box (this call increment the cursor by two). fb.row(); // start a new simple box row fb.add("Primer Apellido: ", surname); // add the first surname text box fb.row(); // start a new simple box row fb.add("Segundo Apellido: ", secondSurname); fb.endBox(); // end simple box fb.row(); // new base component row fb.startBox(); // start a new simple box (note that don't have a fixed height) fb.row(); // new simple box row fb.add(FormUtils.newLabelForBox("Descripción")); fb.row(); // new simpleBox row fb.setHeight(Short.MAX_VALUE); // let this row to be as bigger as it can. fb.add(new JScrollPane(description)); // add the text area fb.endBox(); // end last simple box // build the form return the JComponent. return fb.getForm(); } public static void main(String[] args) { ApplicationContextGuiFactory.setPlasticLookAndFeel(); SampleForm dlg = new SampleForm(); dlg.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE); dlg.setSize(400, 300); dlg.setVisible(true); } }
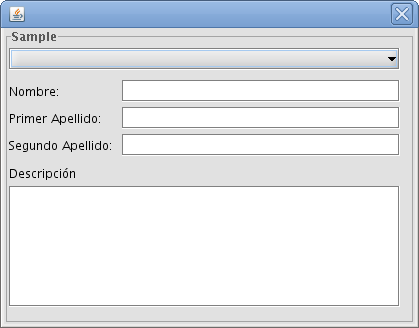